Voxel Destruction
Solo Developer
Dynamic destruction in video games has always fascinated me. The possibility of 100% destructible environments is awesome, and I love games like Rainbow Six: Siege, The Finals, and Battlefield. Over the years, I’ve dabbled with programming my own destruction systems, and my most recent experiment in Unity 6’s Data Oriented Technical Stack (DOTS)🔗 with the Burst Compiler🔗 has been a huge success!
The demo shown above is running on my phone (Samsung Galaxy S23+). Hundreds of physics entities, destruction events every 0.03 seconds, segmentation with an anchoring system, and the framerate is still wonderful! Below, I’ll give a brief overview of the destruction system I developed for this project, as well as how I used DOTS to boost performance.
How It Works
There’s 2 major steps to achieving dynamic destruction:
1. Boolean operations on polygons (Wikipedia🔗)
2. Segmentation
Step 1: Boolean Operations on Polygons
We can simulate causing destruction by cutting a hole in the object we’re destroying. To do this, we have the “positive” and “negative” shapes.
For example: The white rectangle is the positive shape, and the red rectangle is the negative shape.
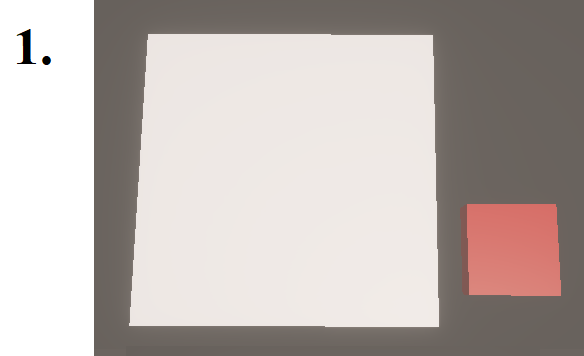
The positive is the shape we’re cutting into, and the negative is the shape we’re cutting. So in the video, the positive is the wall, and the negative is the red bullet hole shape.
Let’s move the negative into the positive:
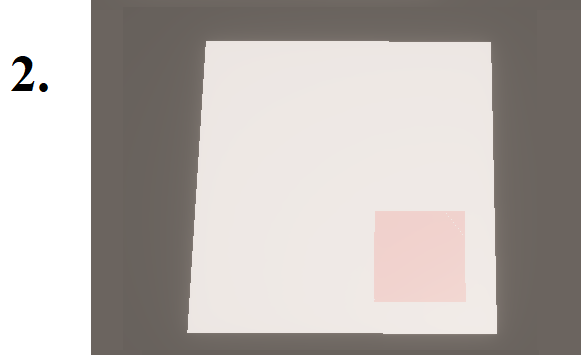
And now let’s perform the boolean operation “Positive NOT Negative.”
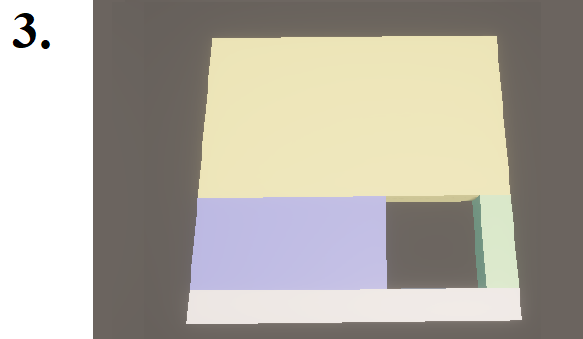
Here we can see that we turned the positive into 4 rectangles. I’ve color coded them so they’re easy to identify.
Here’s the final output:
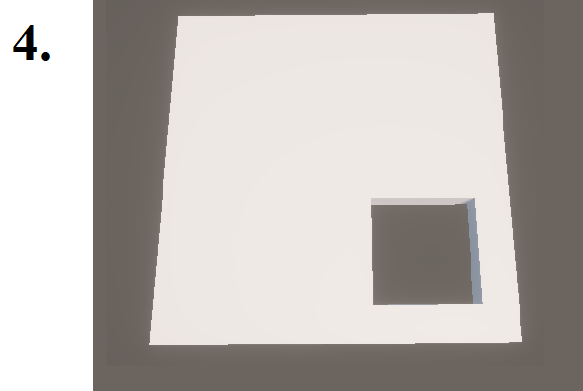
And boom! We just made a hole!
Now, you may be thinking to yourself: “Hey! You just turned one object into 4. That must be inefficient, right?”
As unintuitive as it may be, this is actually great for DOTS. DOTS performs excellently when operating on many of the same object. In our case, we have hundreds of rectangles. Because they’re so similar, they’re contiguous in memory. Hence, DOTS can quickly iterate through all of them, getting great cache prefetching!
Side note: What is cache prefetching?
As a simplified explanation, lets say I have an array: {0,1,2,3,4,5,6,7,8,9}
I iterate this array, getting each value. When I get index 0, the CPU fetches the element at index 0 from main memory, as well as a few elements after index 0. Let’s say it grabbed elements {0,1,2,3}. It places these elements in its cache, a smaller, but faster to access, memory space. Then, when I get index 1, the CPU looks in its cache, sees that it already prefetched that element, and quickly gives it to me. The same thing happens for elements 2 and 3. When I ask for element 4, it fails to find it in cache, and it goes to main memory and gets elements {4,5,6,7}. Etc., etc. By having elements be contiguous in memory, they become cache friendly!
Step 2: Segmentation
By this point, we’ve cut a hole in our shape and ended up with a new shape… or have we? Do we truly have one shape? That’s the interesting question! To a person, the previous example is obviously one shape. However, how do we get a computer to realize this, and how do we get the computer to realize this quickly? The key is to keep track of the rectangles we’ve changed.
For example:
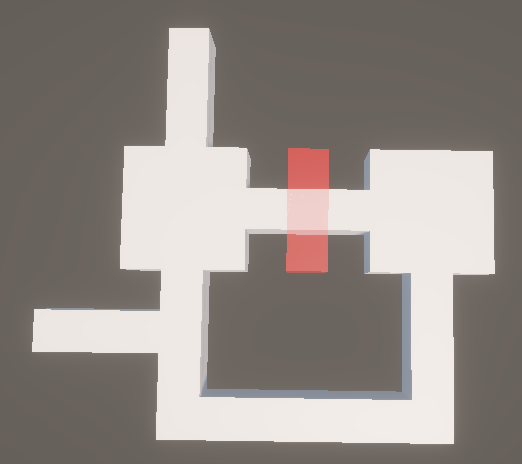
After we perform the boolean operation:
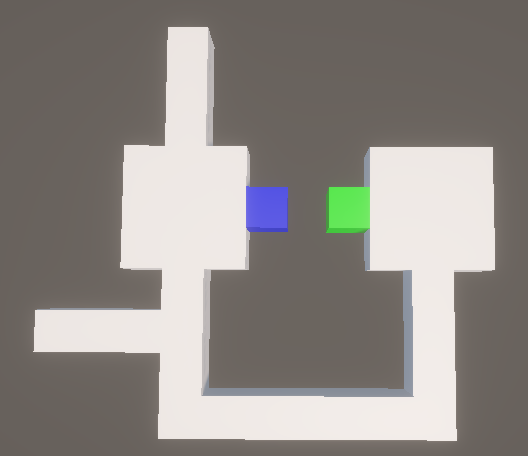
I’ve colored the rectangles that have been modified by the boolean operation. To tell if the shape is still whole, we simply need to check if these 2 shapes are still connected. Unfortunately, they aren’t trivially connected (i.e.: They aren’t directly touching each other). Hence, we need to do some exploration of the shape. Let’s start exploring the rectangles near the blue rectangle, forming a blue group:
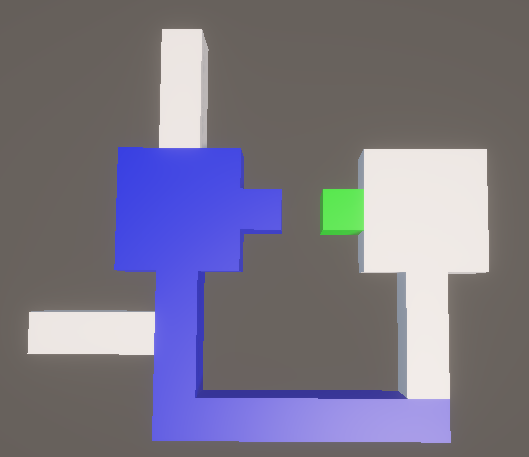
Hmm… we’ve still not found the green group. Let’s try exploring some of the green group:
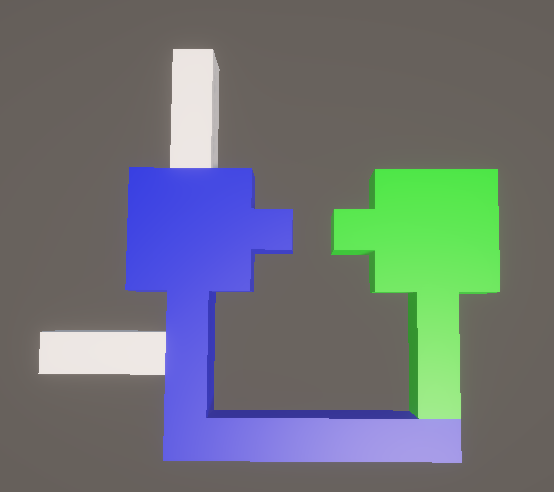
Yay! We’ve found the blue group! Let’s merge.
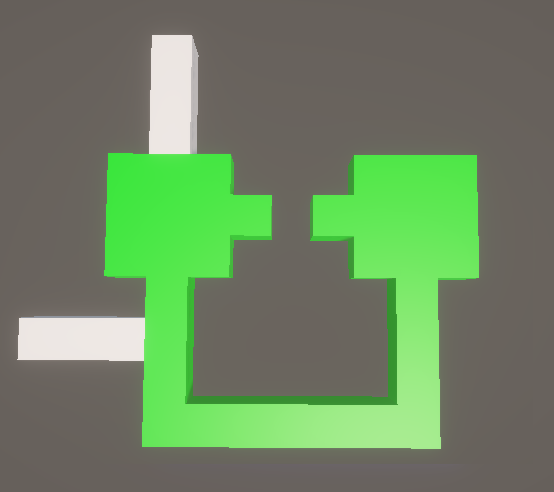
Since we have 1 group, this shape is whole. However, let’s cause more destruction!:
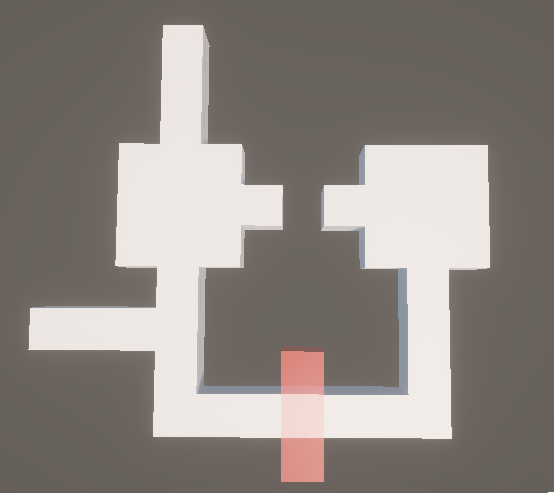
Perform the boolean operation:
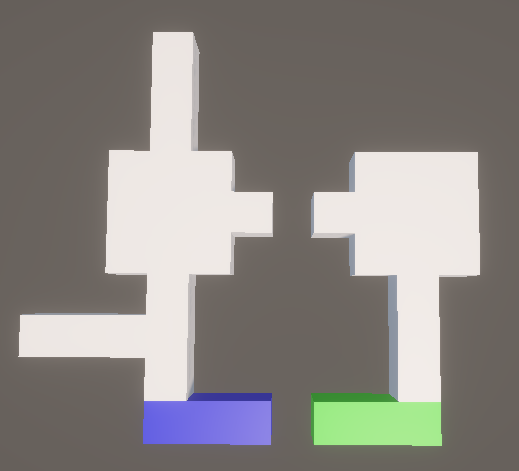
Okay, once again they’re not trivially connected. Let’s explore:
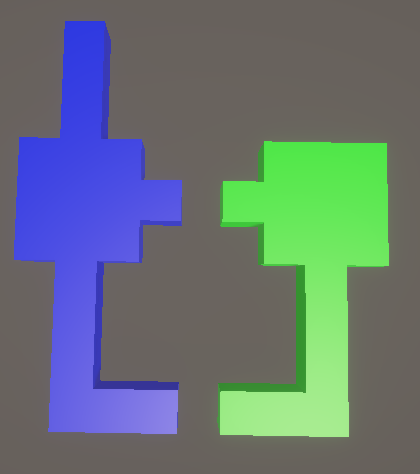
Ta-da! We’ve identified 2 shapes, so we’ll create separate entities for each. That’s everything we need for basic destruction. However, how do we achieve something a bit… more?
These are all rectangular prisms. I’d like voxels! The solution: Shaders!
Shaders
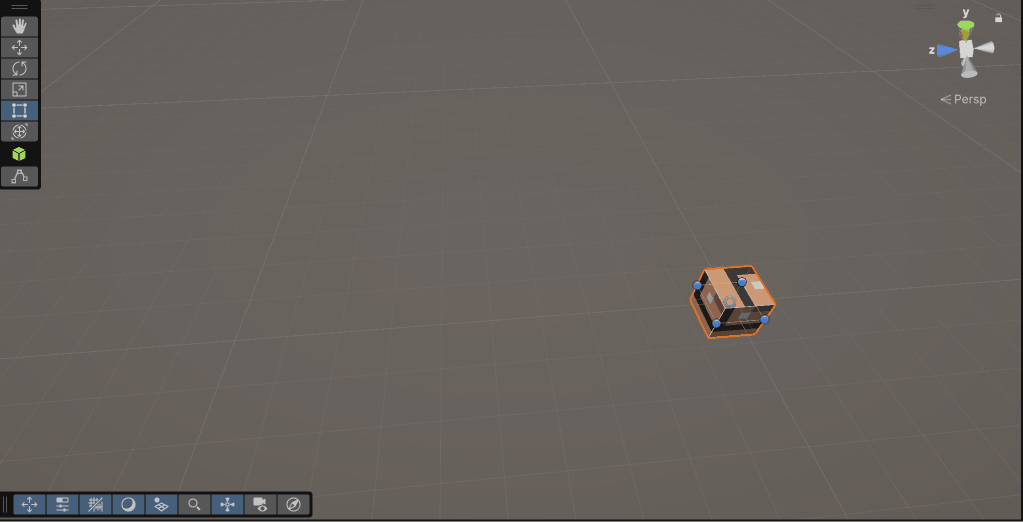
By using a shader that maps UV’s to world space, we can make it look like we’re working in voxels, even when we’re working in, far more efficient, rectangular prisms!
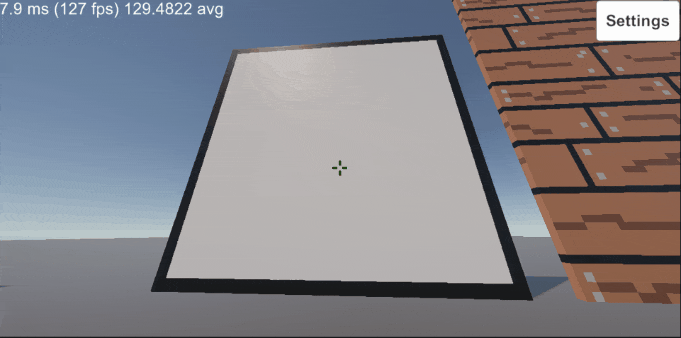
Here, I’ve disabled the shader so you can see the rectangular prisms.
The Final Result
Now, this is a simplified explanation of my destruction system, and it’s quite a bit more complex than this. However, hopefully you have a general idea of how this works. If we ever bump into each other, feel free to ask me (Leon Milewski🔗) all about it! I love talking dynamic destruction systems!